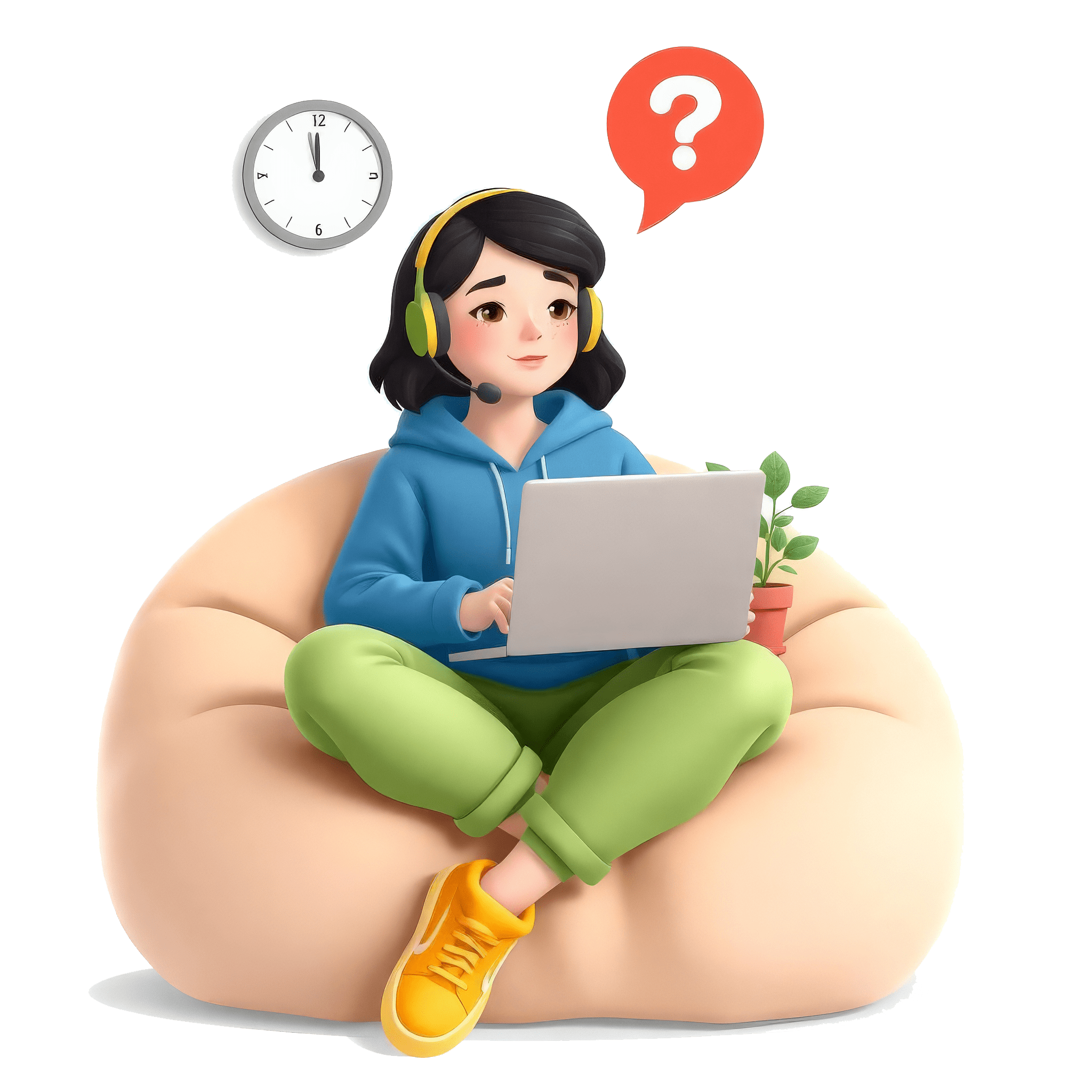
.NET Code Challenges & Tips
- 10 Topics
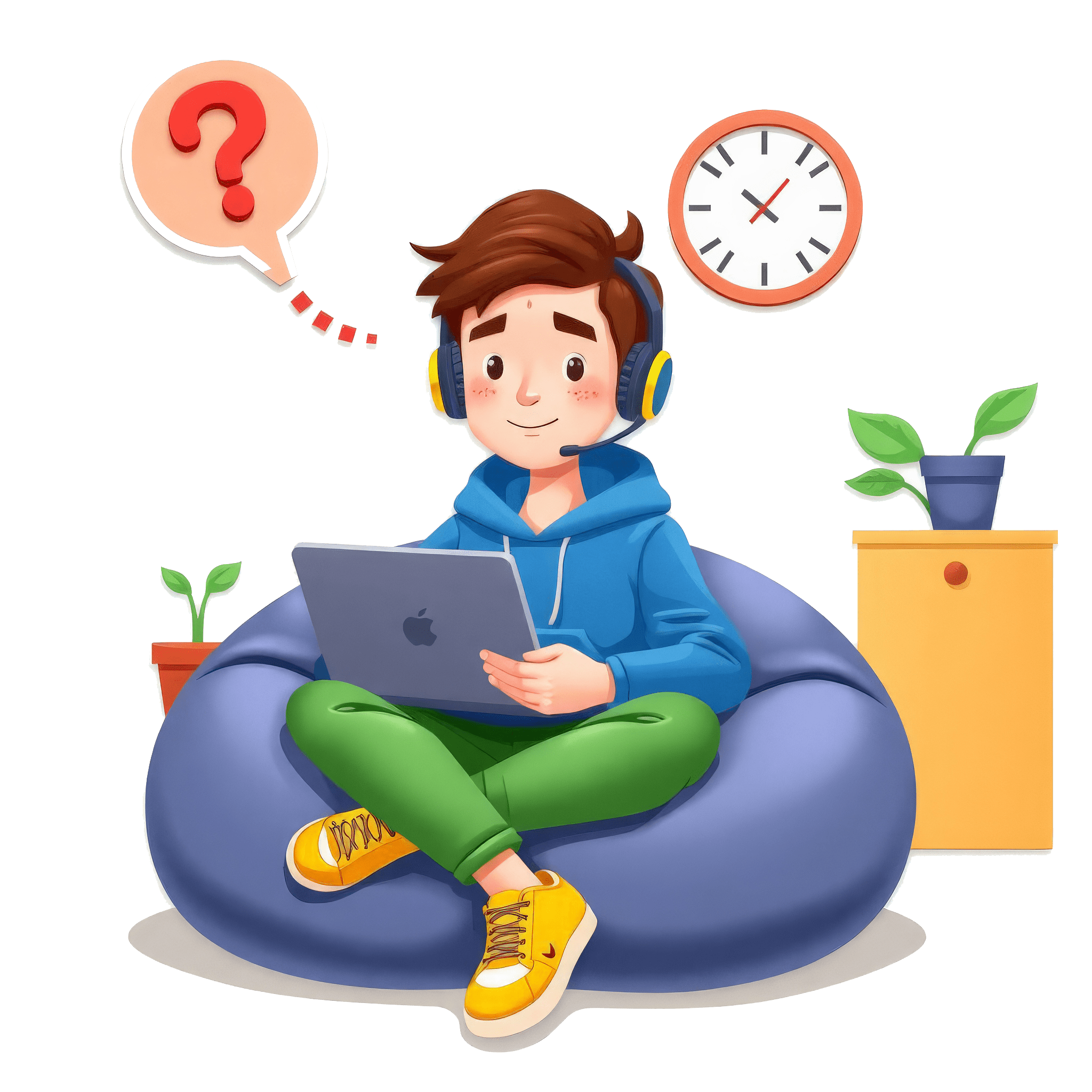
1. Write a function in C# to determine if a given string is a palindrome, ignoring spaces, punctuation, and capitalization.
2. Implement a method that takes two sorted lists and returns a new sorted list that contains all elements from both lists without duplicates.
3. Create a function that takes an integer array and returns the maximum sum of any contiguous subarray (Kadane’s Algorithm).
4. Write a recursive function to calculate the nth Fibonacci number.
5. Implement a class that represents a basic calculator capable of performing addition, subtraction, multiplication, and division operations based on an input string in Reverse Polish Notation.
6. Write a method to find the longest common prefix string amongst an array of strings. If there is no common prefix, return an empty string.
7. Create a function that merges two binary trees into a new binary tree by adding values at overlapping nodes.
8. Write a method that finds the first non-repeating character in a string and returns its index. If it doesn't exist, return -1.
9. Implement a function to check if a given string has all unique characters without using any additional data structures.
10. Write a function that takes a string as input and returns all possible subsets (the power set) of that string.
Reveal all the approved answers to the questions above and explore all the types of .NET questions and answers.
Explore Now