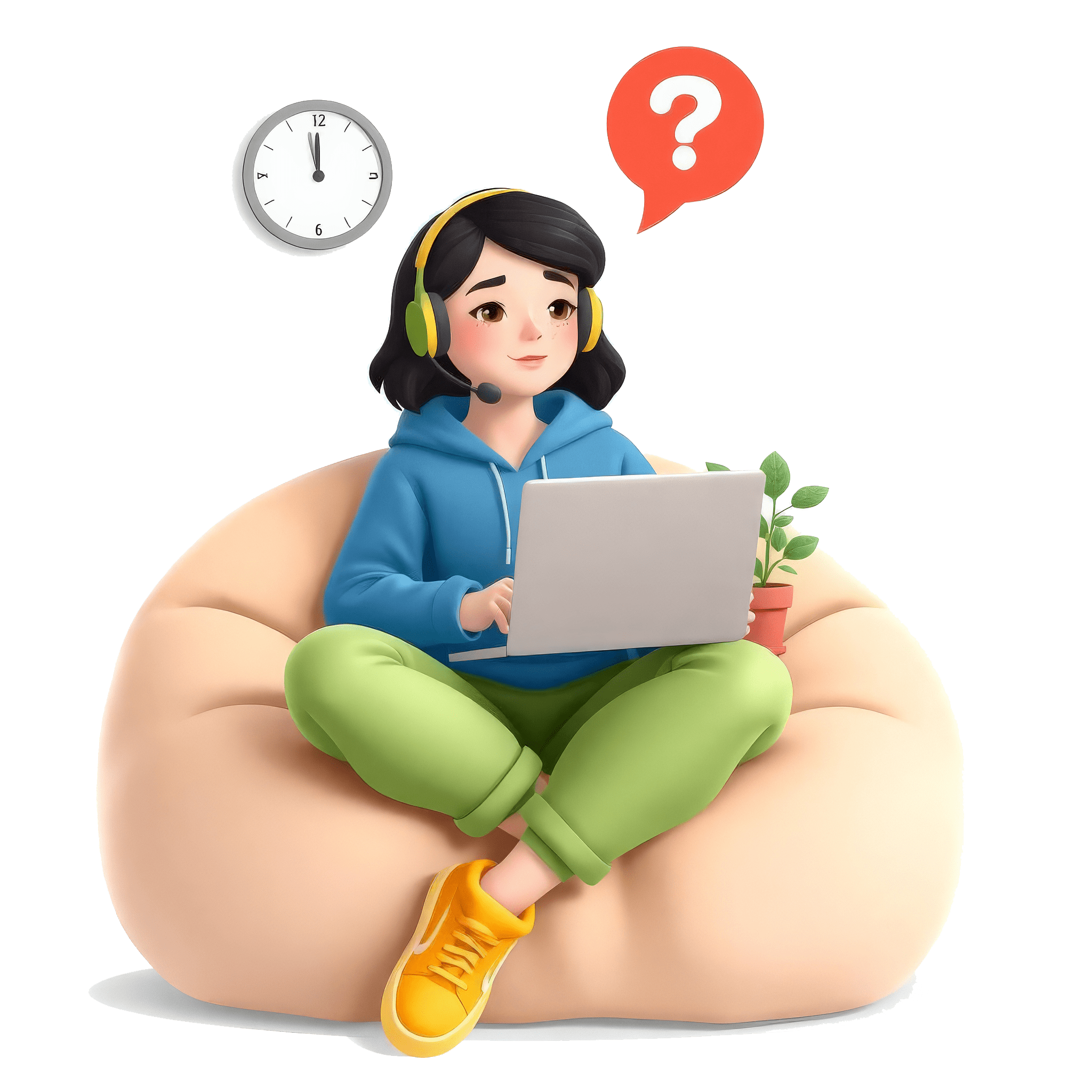
Java Spring Boot Code Challenges & Tips
- 10 Topics
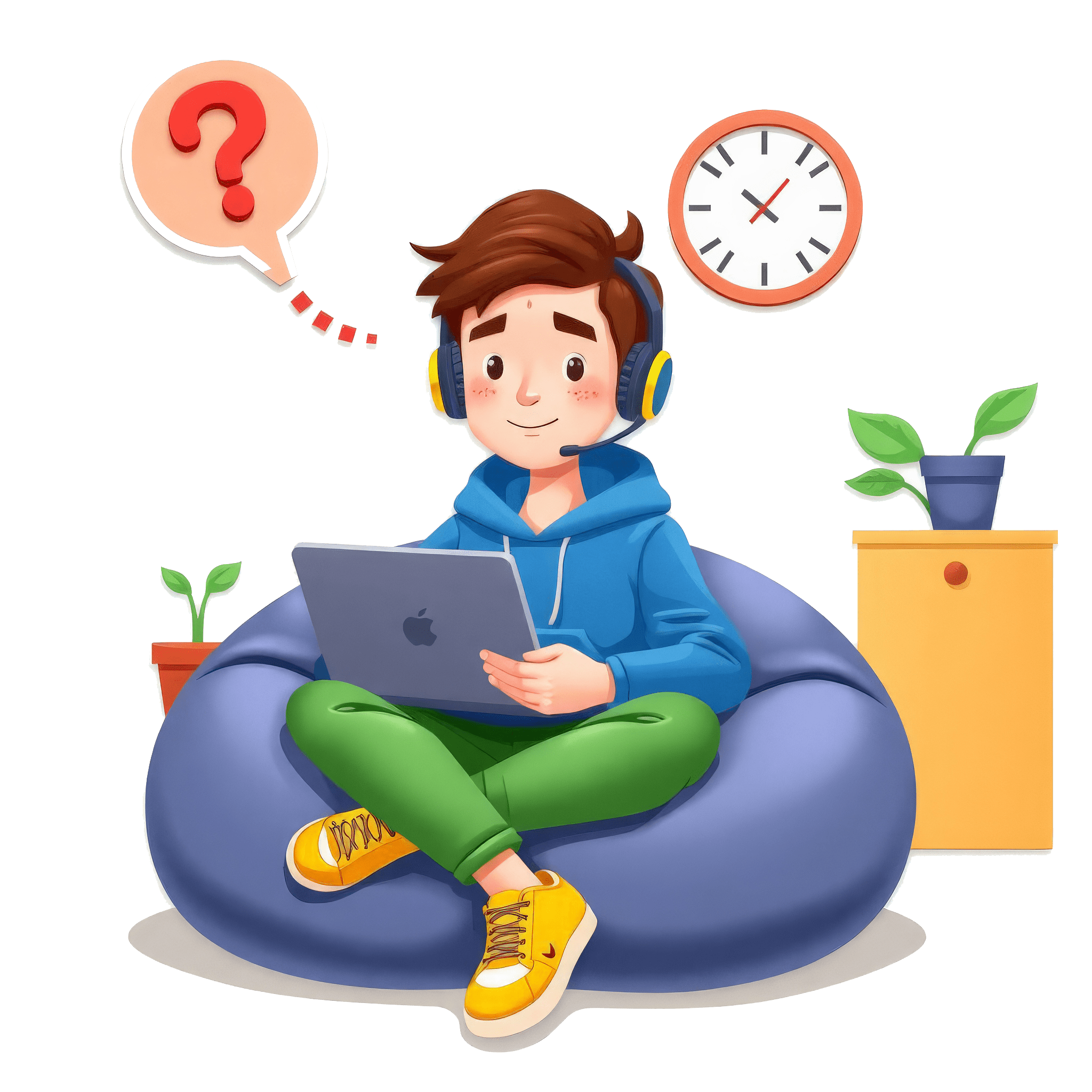
1. Write a Spring Boot REST controller that handles CRUD operations for a `User` entity with fields `id`, `name`, and `email`.
2. Implement a Spring Boot service that asynchronously retrieves data from an external API and returns it to the caller, utilizing `CompletableFuture`.
3. Create a Spring Boot application that uses JPA to connect to a relational database, and implement a method to perform a paginated search on a `Product` entity based on name and category.
4. Write a method to create a custom Spring Security filter that authenticates a user using JWT and restricts access to certain endpoints based on user roles.
5. Implement a Spring Batch job that reads data from a CSV file, processes it, and writes the results to a PostgreSQL database.
6. Develop a service in Spring Boot that implements caching using Spring’s Cache abstraction. The service should include methods to add, retrieve, and evict cached data.
7. Given a `List<String>`, write a Spring Boot controller that returns the most frequent string along with its count in a JSON response.
8. Write a Spring Boot application that integrates with an external message broker (like RabbitMQ or Kafka) to create a simple producer-consumer messaging system.
9. Create a Spring Boot application that uses Aspect-Oriented Programming (AOP) to log execution time of service methods. Write an aspect to achieve this.
10. Implement a method in a Spring Boot application that converts a given object to JSON and sends it as a response with the appropriate HTTP status code.
Reveal all the approved answers to the questions above and explore all the types of Java Spring Boot questions and answers.
Explore Now