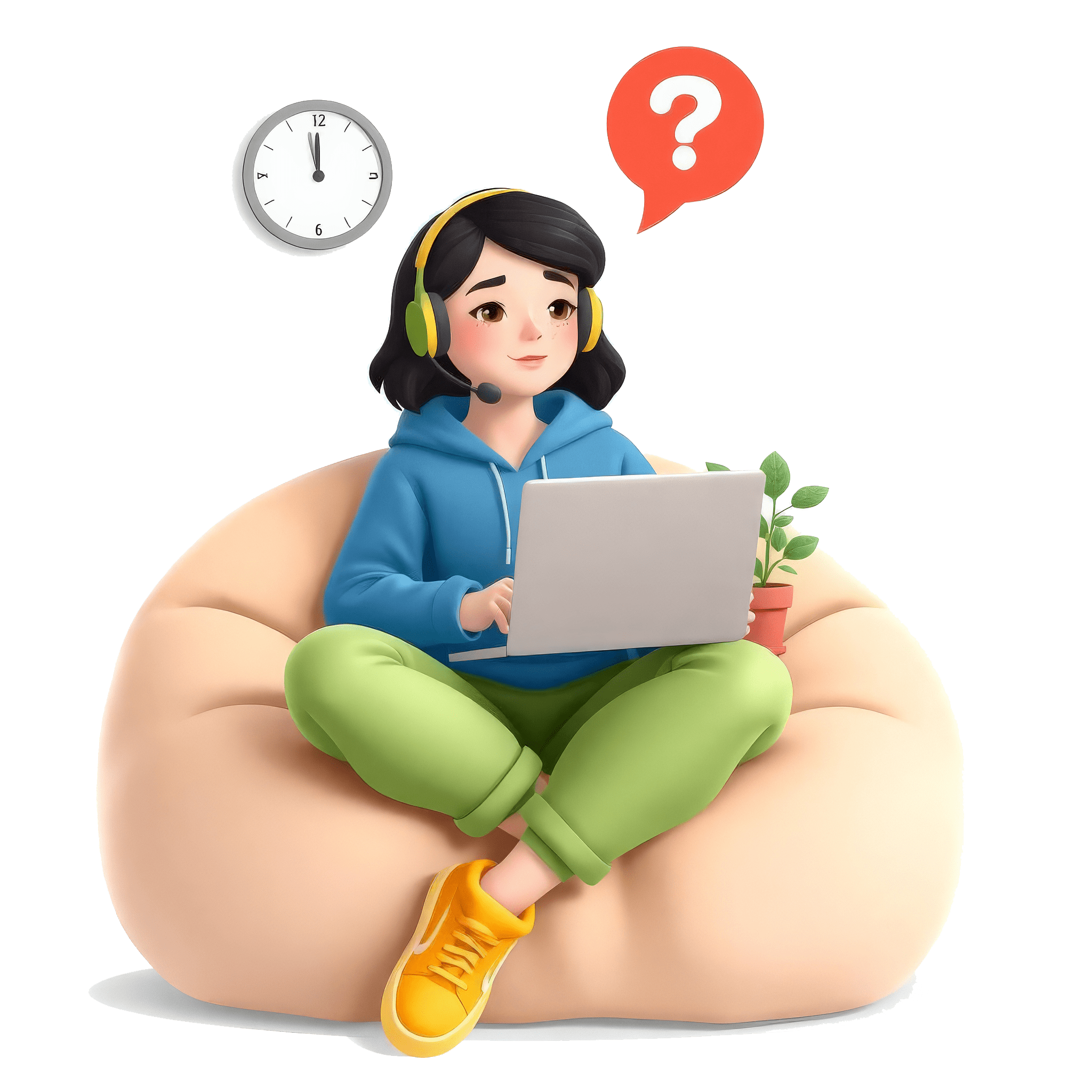
Android Problem-Solving and Analytical Questions
- 10 Topics
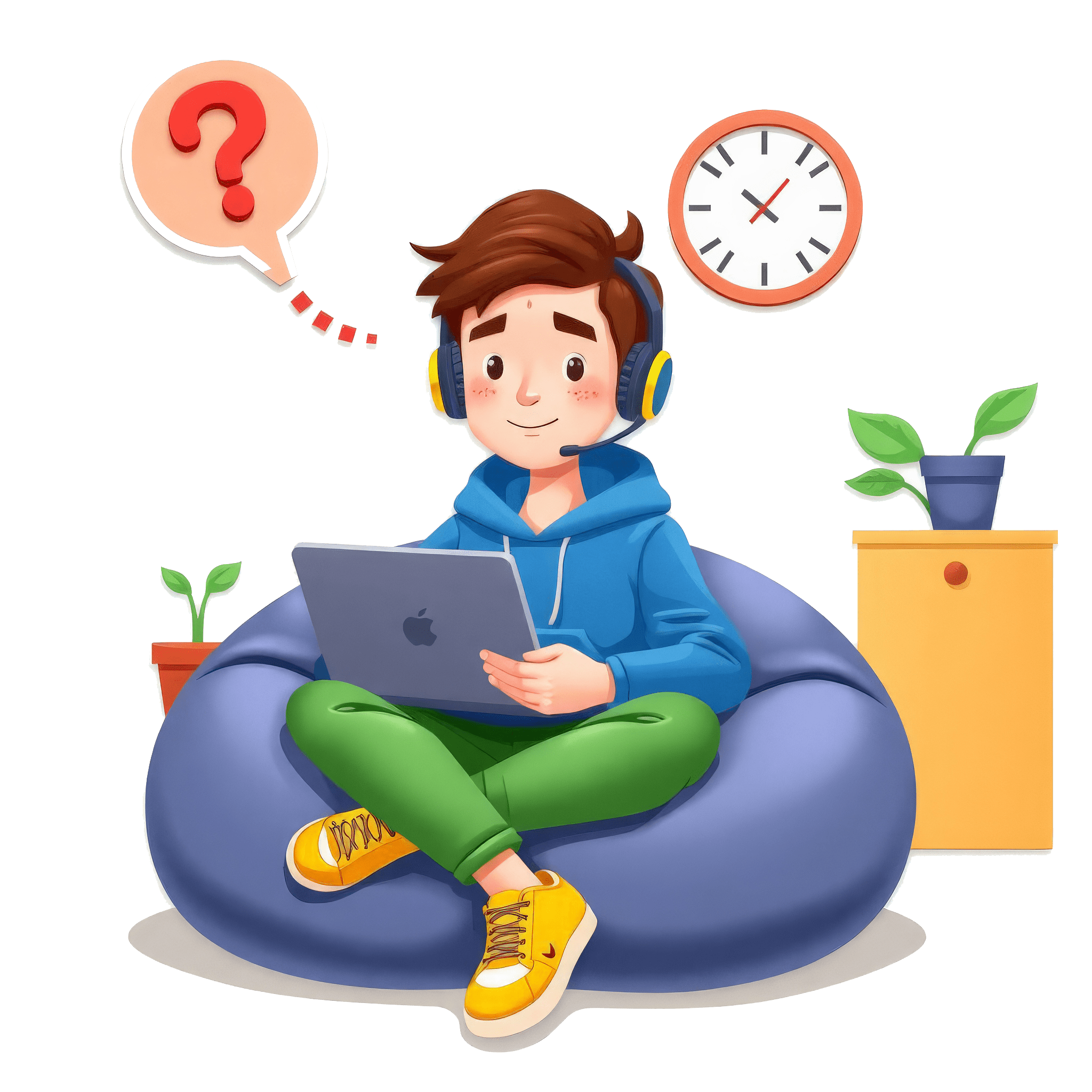
1. You have a list of integers that can appear multiple times. Write an algorithm to find the most frequently occurring integer. If there are ties, return the smallest integer.
2. Given a string, write a function to determine if it is a permutation of a palindrome.
3. You have a binary tree. How would you check whether it is a binary search tree (BST) or not?
4. Describe how you would implement a Least Recently Used (LRU) cache using Android's memory management features.
5. A professor is grading all of his students' exams. He has a list of the scores (out of 100) and wants to know how the average score would change if he removed the highest and lowest scores. Write a method that calculates the new average.
6. Write a function that takes a list of numbers and returns the maximum product of any three numbers.
7. An Android app needs to retrieve data from a remote server and cache it locally. Describe how you would design this caching system, considering scenarios of data update, retrieval, and storage efficiency.
8. You're tasked to find the longest substring without repeating characters in a given string. Describe the algorithm you would use, considering both time and space complexity.
9. You have an array of integers where each element represents the maximum number of steps you can take forward from that element. Write an algorithm to determine whether you can reach the last index.
10. Given a set of GPS coordinates for various locations, implement an algorithm that finds the two closest points to each other.
Reveal all the approved answers to the questions above and explore all the types of Android questions and answers.
Explore Now