- October 18, 2024
- 5 min read
- 1
- 1K
The Ultimate Guide to C++ Interview Preparation
C++ Developers & Programmers: Your Ultimate Guide to Interview Preparation
When it comes to securing a software development position, especially in C++, mastering data structures and algorithms is crucial. For candidates preparing for interviews, a robust understanding of both C++ and the complexities of data structures and algorithms can be the difference between landing that job and missing out. This guide will shine a light on effective strategies, important topics to cover, and how to prepare to ace your interviews.Why C++?
C++ remains a cornerstone in the programming world, utilized in systems programming, game development, applications requiring high performance, and more. Being well-versed in C++ not only increases employability but also equips programmers with intuitive problem-solving skills.Understanding Data Structures and Algorithms
Data structures are ways of organizing and storing data, while algorithms are the procedures for processing that data. Key data structures you should master include:- Arrays: Basic structures used for storing data in a linear format. Understanding how to manipulate arrays is fundamental in C++.
- Linked Lists: A dynamic data structure that consists of a sequence of nodes. Mastering linked lists allows for better memory management.
- Stacks and Queues: These are essential for scenarios requiring LIFO (Last In First Out) and FIFO (First In First Out) operations, respectively.
- Trees: Understanding binary trees, binary search trees, and balanced trees (like AVL and Red-Black Trees) is critical for hierarchical data storage and retrieval.
- Graphs: Essential for understanding more complex data connectivity and relationships. Familiarity with traversals (BFS and DFS) is vital.
Core Algorithms to Master
A good grasp of algorithms can immensely boost your problem-solving skills. Here are some essential algorithm categories:- Sorting Algorithms: Get comfortable with QuickSort, MergeSort, and BubbleSort. Understanding time and space complexities is key.
- Searching Algorithms: Binary search, Linear search, and understanding how to work with sorted data can be a recurrent question in interviews.
- Dynamic Programming: This advanced topic involves breaking down problems into simpler subproblems. It’s essential for optimization tasks.
- Recursion: Mastering recursion will help in solving problems related to trees and backtracking.
Interview Preparation Strategies
1. Solve Real Problems: Websites like [Interview Plus](https://www.interviewplus.ai/developers-and-programmers/c-plus-plus/questions) compile questions frequently asked in interviews. Practicing real interview questions gives you a feel of what to expect.
2. Study Patterns: Identify common problem patterns, such as two-pointer techniques or sliding window problems. Recognizing these patterns can speed up your response time in interviews.
3. Mock Interviews: Conduct mock interviews with peers or utilize platforms where you can practice coding under time constraints. This will help reduce anxiety during the actual interview.
4. Understand Complexity: Be prepared to discuss the time and space complexity of your solutions. Interviewers often seek candidates who can not only provide solutions but also articulate their efficiency.
5. Revise Fundamentals: Revisiting the fundamentals of C++, including its standard libraries (STL), object-oriented programming concepts, and memory management, is crucial.
Key Concepts in C++
- Pointers and References: Understand how pointers work, along with memory allocation and deallocation (new and delete).
- Object-Oriented Programming: Concepts such as inheritance, polymorphism, encapsulation, and abstraction must be second nature to you.
- Templates and Exception Handling: Be prepared to discuss generic programming and how exceptions are handled in C++.
Final Thoughts
The key to success in C++ interviews lies in solidifying your understanding of data structures and algorithms, coupled with hands-on practice. As you prepare for your interviews, focus on both practical coding skills and theoretical knowledge. The programmer's journey is continuous; every interview is an opportunity to learn.Remember, consistent practice is critical to mastering these skills. Leverage coding interview platforms, engage with coding communities, and challenge yourself with new problems. With determination and thorough preparation, you can excel in your C++ programming interviews and secure that desired position in tech!Practice interviews now and evaluate realtime?
Try NowTags:
Other blogs you might be interested in:
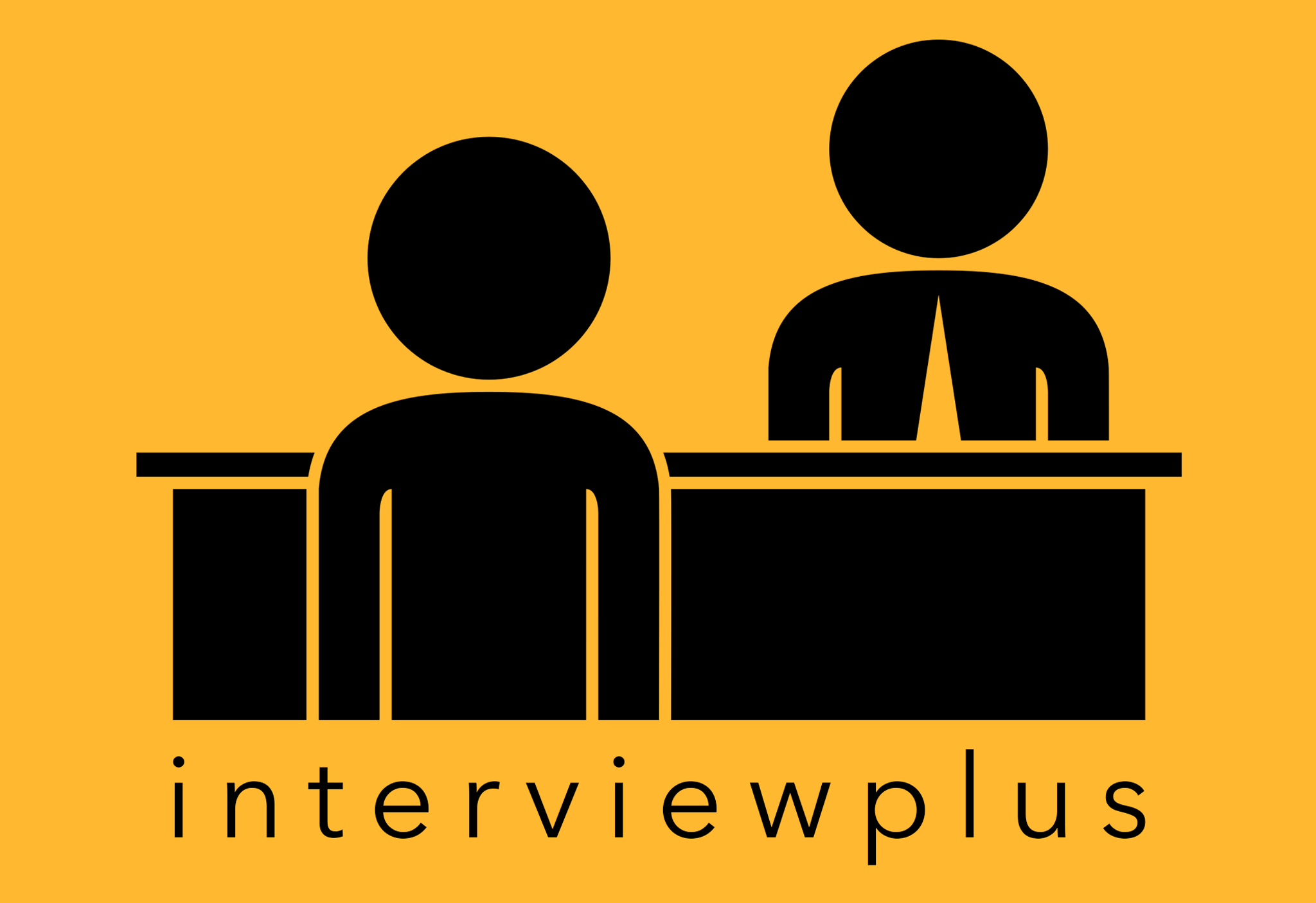
Essential Interview Questions for Junior Environmental Scientists
Prepare for your Junior Environmental Scientist interview with key questions and insights to succeed in your environmental career.
Interviewplus
October 21, 2024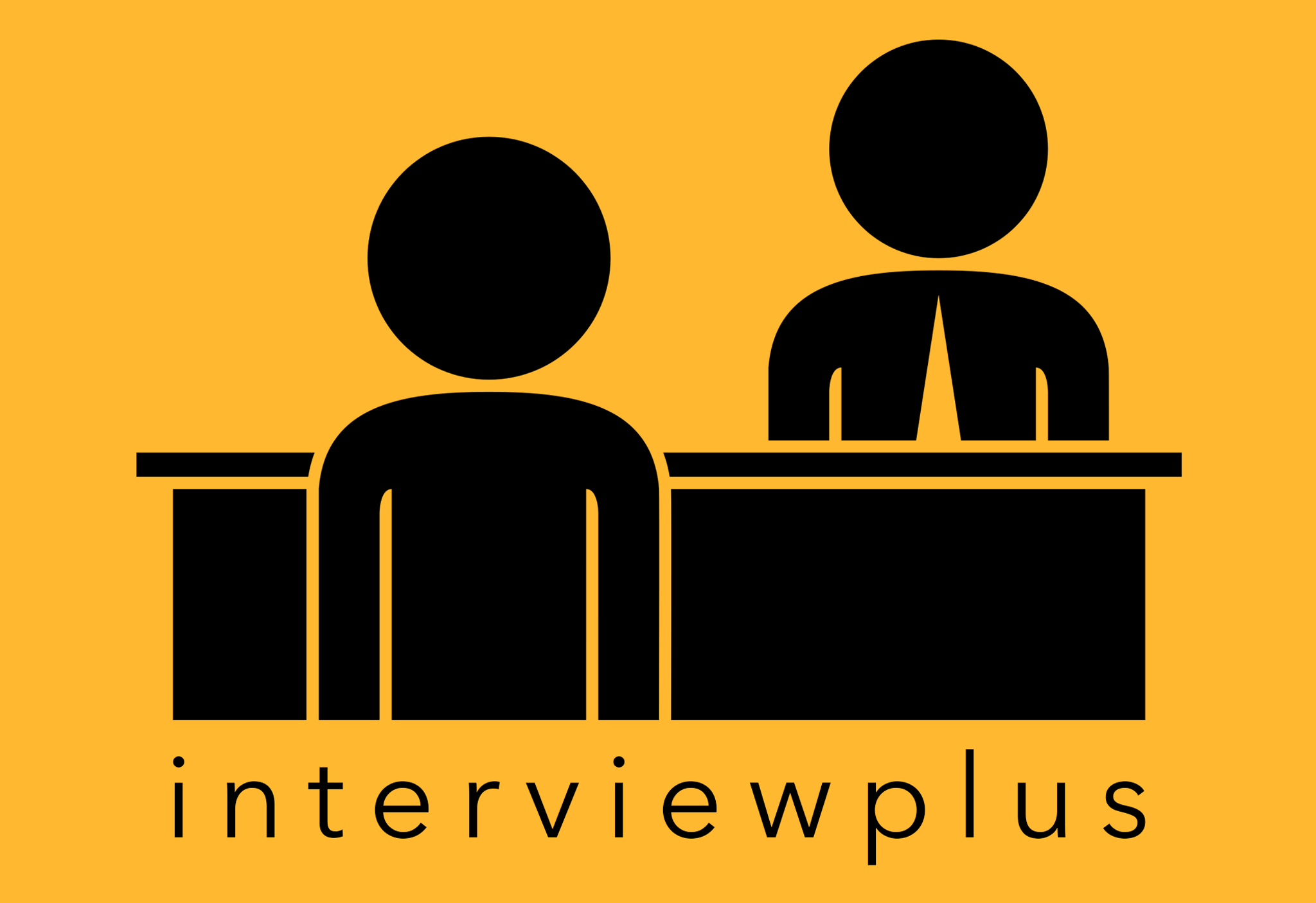
The Ultimate Guide to Chef Skill Assessment Questions
Prepare for Chef skill assessment interviews with this comprehensive guide, featuring essential concepts and valuable interview questions.
Interviewplus
December 15, 2024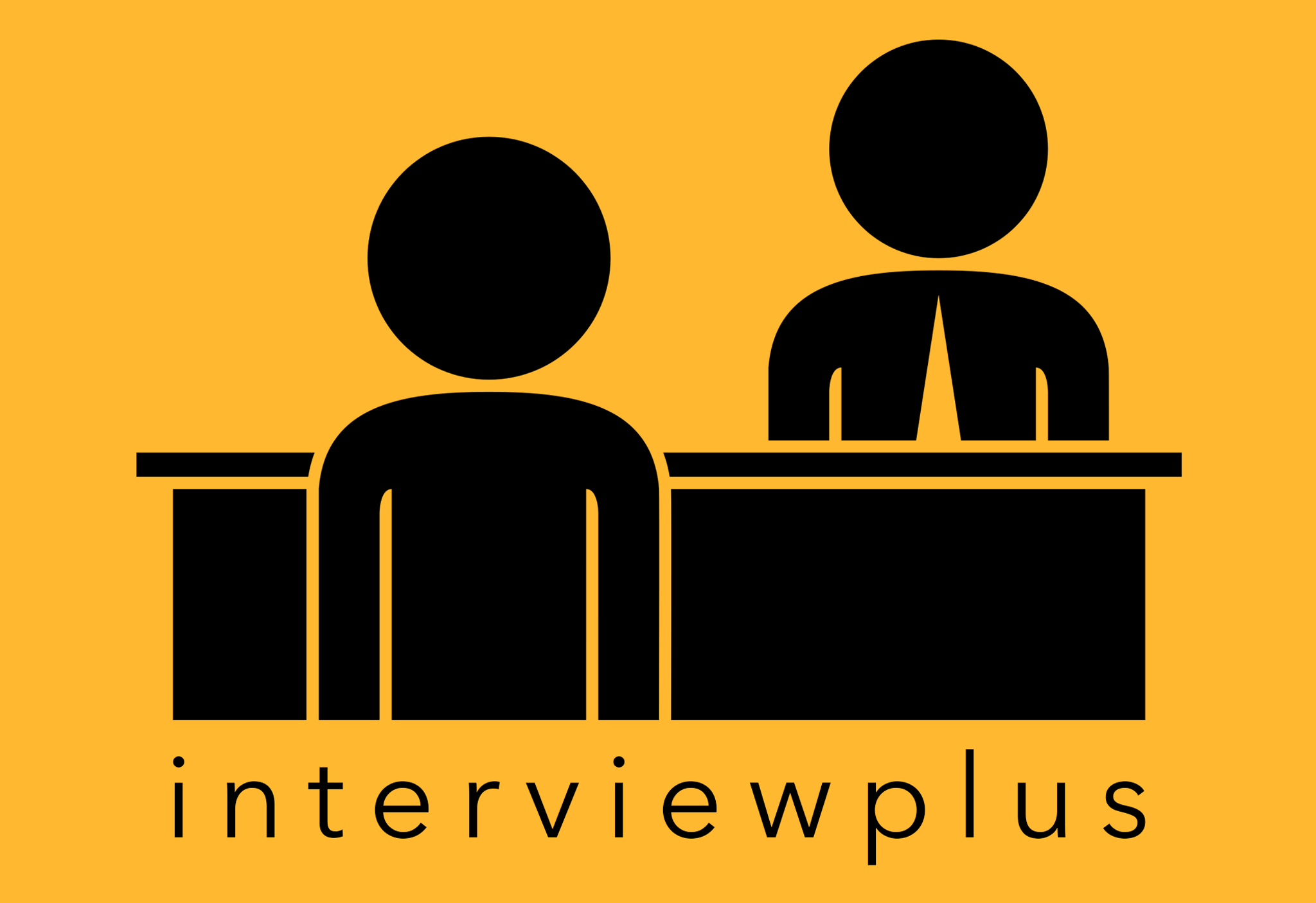
The Ultimate Guide to Climate and Energy Manager Interviews
Master the art of interviewing for a Climate and Energy Manager role with essential questions and tips for success. Be prepared and get hired!
Interviewplus
November 11, 2024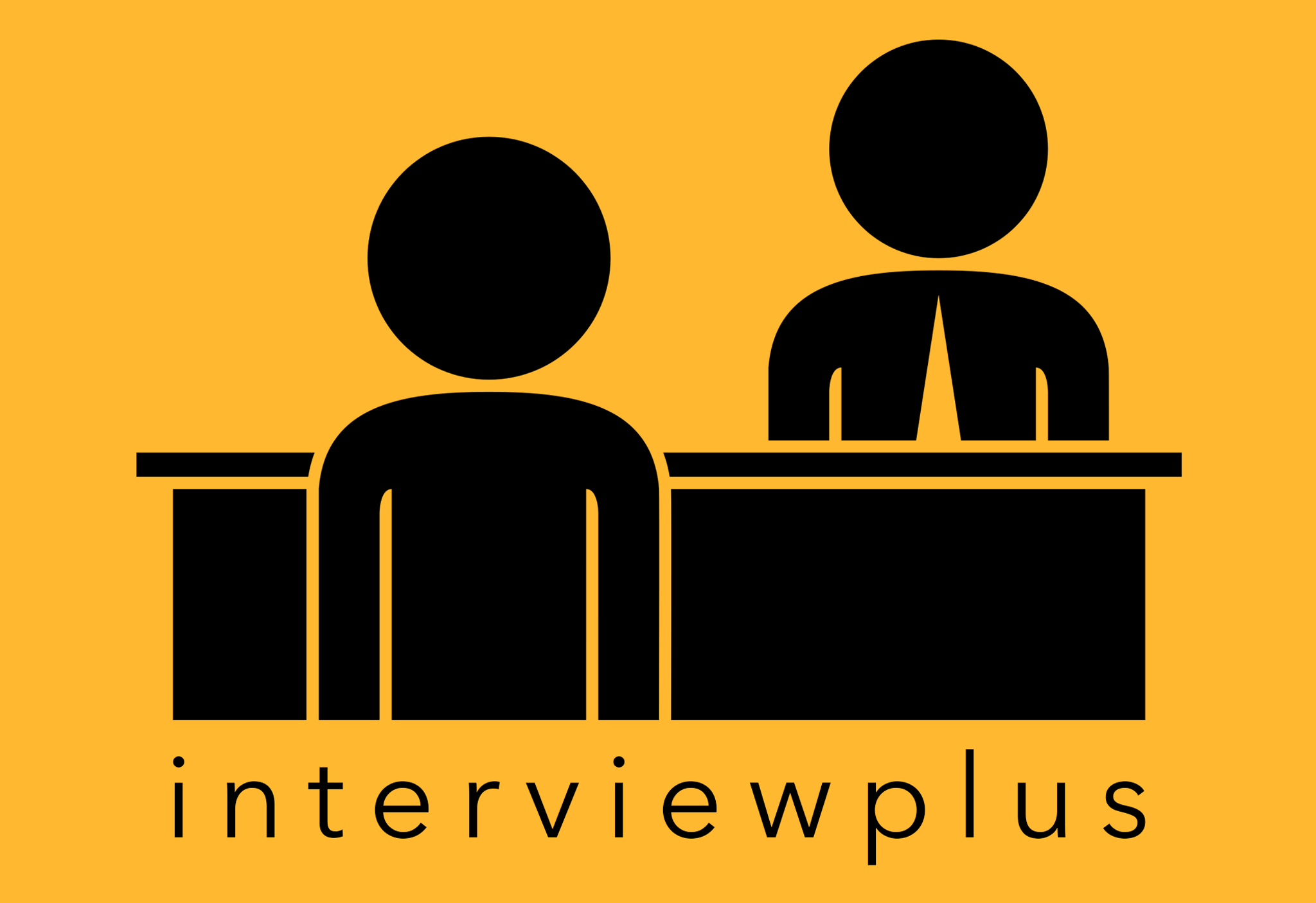
Everything You Need to Know About Project Manager Interviews
Prepare effectively with essential project manager interview questions and tips for success. Boost your chances and land your dream job!