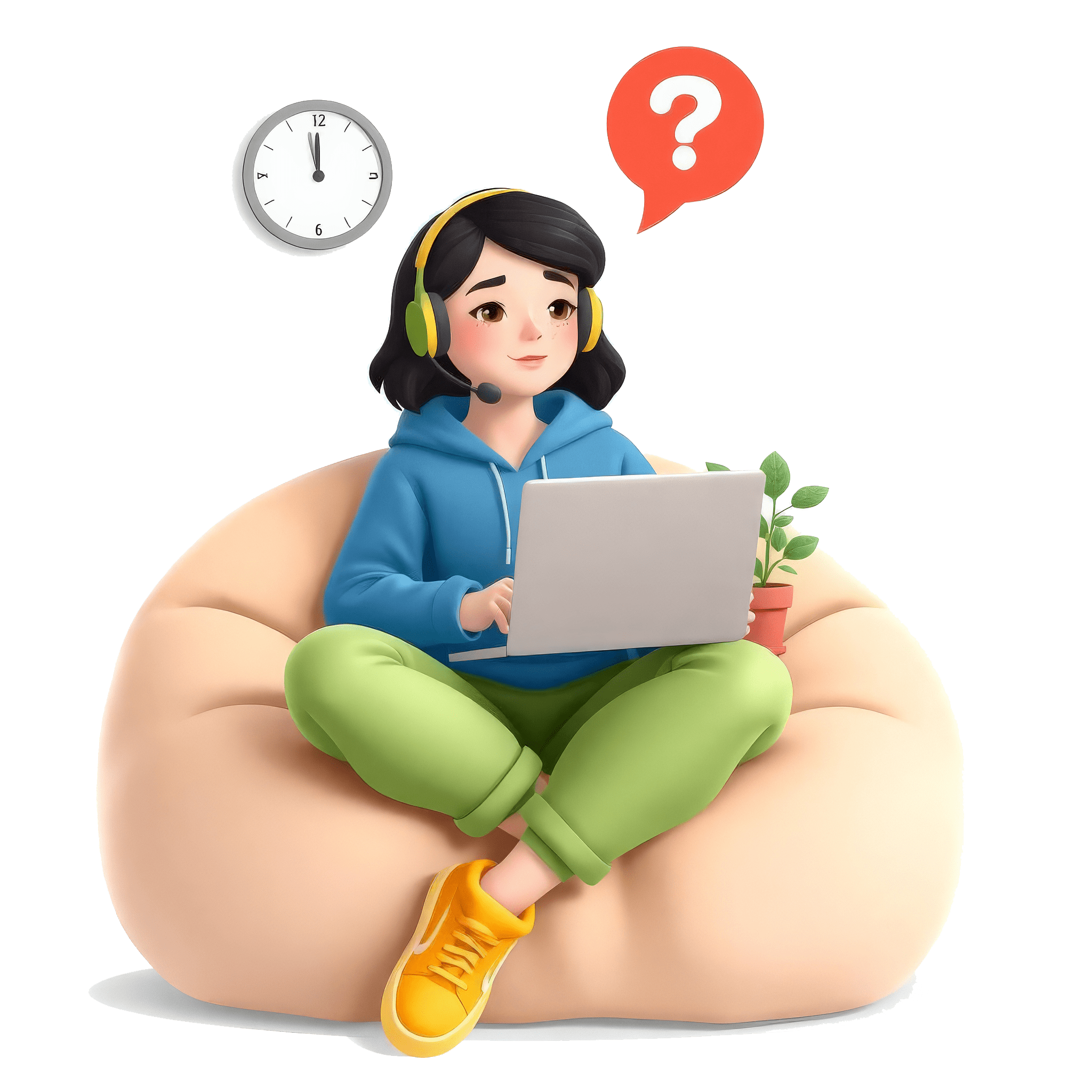
Developers & Programmers Interview
C++ Question(s) & Actual Evaluation
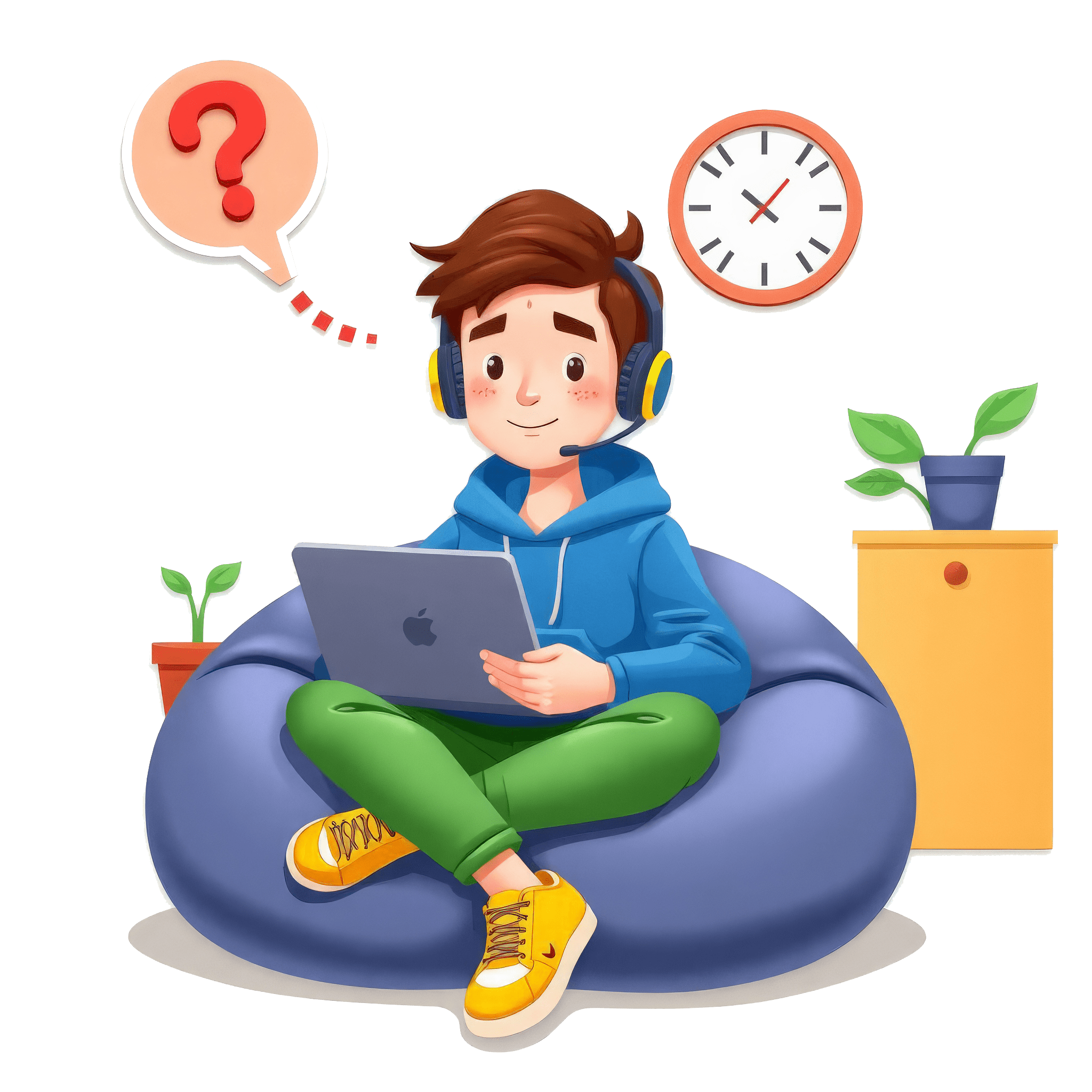
Please refer to the evaluation for more insight.
-
Classification: Developers & Programmers
-
Topic(s): C++
Can you explain the difference between a pointer and a reference in C++? When would you use one over the other?
Candidate's Answer:Pointer stores the address of the variable and * is used to pointer.& operator is used for refernce and it is used to find the value that address contains
Evaluation:Your answer provides a basic understanding of pointers and references in C++, but it lacks clarity and depth in some areas. Here's an evaluation:
1. Correct Points: You mentioned that pointers store addresses and that the `*` operator is used with pointers. However, the explanation about references is somewhat unclear; the `&` operator is used to declare a reference, not to find the value that the address contains.
2. Depth of Explanation: Your answer could benefit from elaborating on key concepts, such as:
- Pointers: They can be reassigned to point to different addresses, can be null, and must be dereferenced using `*` to access their value.
- References: They must be initialized upon declaration and cannot be changed to reference another variable later, serving more like an alias to the original variable.
3. Use Cases: Mentioning scenarios where each is preferable could strengthen your answer. For example:
- Use pointers when you need to manage dynamic memory or when you need to support null values.
- Use references for function parameters and return types when you want to avoid copying large objects and ensure an aliasing behavior.
Improved Answer Example:
"Pointers in C++ store the memory address of a variable and can be reassigned to different addresses, making them very flexible but also requiring careful management, particularly with memory allocation. The `*` operator is used to dereference a pointer to access the value at the address. References, on the other hand, act as an alias to a variable and must be initialized when declared. They cannot be reassigned, which makes them safer to use. References are typically preferred for function parameters when you want to avoid copying large objects and maintain the original data. Pointers are useful when you require more control, such as dynamic memory management or when a null pointer is a valid state."
Rating: 3/5. Your answer contained key concepts but lacked clarity and depth, and did not fully address usage scenarios. There’s room for improvement in structured responses and technical details.
-
Classification: Developers & Programmers
-
Topic(s): C++
What are the main principles of Object-Oriented Programming? Can you give examples of how you have applied these principles in your projects?
Candidate's Answer:There are 4 main principles in object oriented program. First one is abstraction, encapsulation, polymerism and inheritance.We use abstraction for hiding of complex implementation and encapsulation for hiding of data polymorphism is used. Polymorphism is nothing but the ability of object to act differently under different conditions and by using inheritance we can.Use properties and behavior of the parent class in subclasses. Suggest derived classes.
Evaluation:Your answer covers the main principles of Object-Oriented Programming (OOP) but requires some refinement and clarity. You identified the four core principles: abstraction, encapsulation, polymorphism, and inheritance. However, the explanations were somewhat vague and could be improved for better understanding.
1. Abstraction: You should emphasize that abstraction simplifies complex systems by exposing only essential features while hiding implementation details. An example would be a user interface for a car where the user doesn't need to know how the engine works to drive it.
2. Encapsulation: While you mentioned hiding data, clarify that encapsulation involves bundling data and methods that operate on it within a single unit or class, restricting direct access to some components. This protects the integrity of the data.
3. Polymorphism: It’s important to specify that polymorphism allows methods to do different things based on the object that it is acting upon, enabling a single interface for different data types. Examples include method overriding and method overloading.
4. Inheritance: You've mentioned property inheritance, but you could elaborate that it allows a new class (derived class) to inherit attributes and methods from an existing class (base class), promoting code reuse and establishing a hierarchical relationship.
To improve your answer, consider adding specific examples from your projects demonstrating the application of these principles. For instance, discuss a project where you created a class hierarchy using inheritance, or how you implemented encapsulation by restricting access to certain class members.
Rating: 3/5.
-
Classification: Developers & Programmers
-
Topic(s): C++
How does C++ handle memory management? Can you describe what "RAII" (Resource Acquisition Is Initialization) means?
Candidate's Answer:C handles memory management through both manual and automatic.Math Mechanisms.C allows developers allocate.And do you look at memory manually using operators like new and delete? New allocates memory on the heap and returns a pointer, while delete releases that memory developers are responsible for matching every new with a corresponding delete to our memorial leaks.Variables are located on stack are automatically managed by compiler. When a variable goes out of scope, the memory is automatically reclaimed without manual intervention and REI resource acquisition is initializing. RAA is a core C++ concept that helps.Sources more safely and efficiently. With REI, a resource is acquired and acquired and managed by an object. The resource is initialized when the object is created and released when the object goes out of scope. For instance, if an object manages a dynamic memory, it will handle the allocation in its construct.And deallocation by its destructor.
Evaluation:Your answer covers several key aspects of memory management in C++ and touches upon RAII, but it could be clearer and more structured. Here’s an evaluation of your answer:
1. Clarity and Structure: Your answer is somewhat disorganized and contains some grammatical issues, which can make it harder to follow. For instance, the starting point about C could be less relevant since the focus was on C++. A clearer separation between manual and automatic memory management, as well as a more straightforward explanation of RAII, would enhance understanding.
2. Detail Accuracy: You correctly mention how `new` and `delete` are used for manual memory management in C++, and how stack variables are automatically managed. However, RAII should be articulated more formally. It’s not just about acquiring resources but ensuring that resources are released automatically when they go out of scope.
3. Examples: It would strengthen your explanation to include a concrete code example that demonstrates RAII, such as a smart pointer like `std::unique_ptr` or a custom class that manages resource allocation and deallocation.
To improve your answer, consider restructuring it to:
1. Briefly define memory management in C++.
2. Explain the difference between stack and heap memory management.
3. Define RAII more clearly and give an example of it in practice.
4. Conclude with the benefits of RAII in preventing memory leaks and enhancing resource safety.
Based on the clarity, relevance, and completeness of your response, I would rate your answer 3/5. Enhance your structure, clarity, and include specific examples to improve your responses in future interviews.