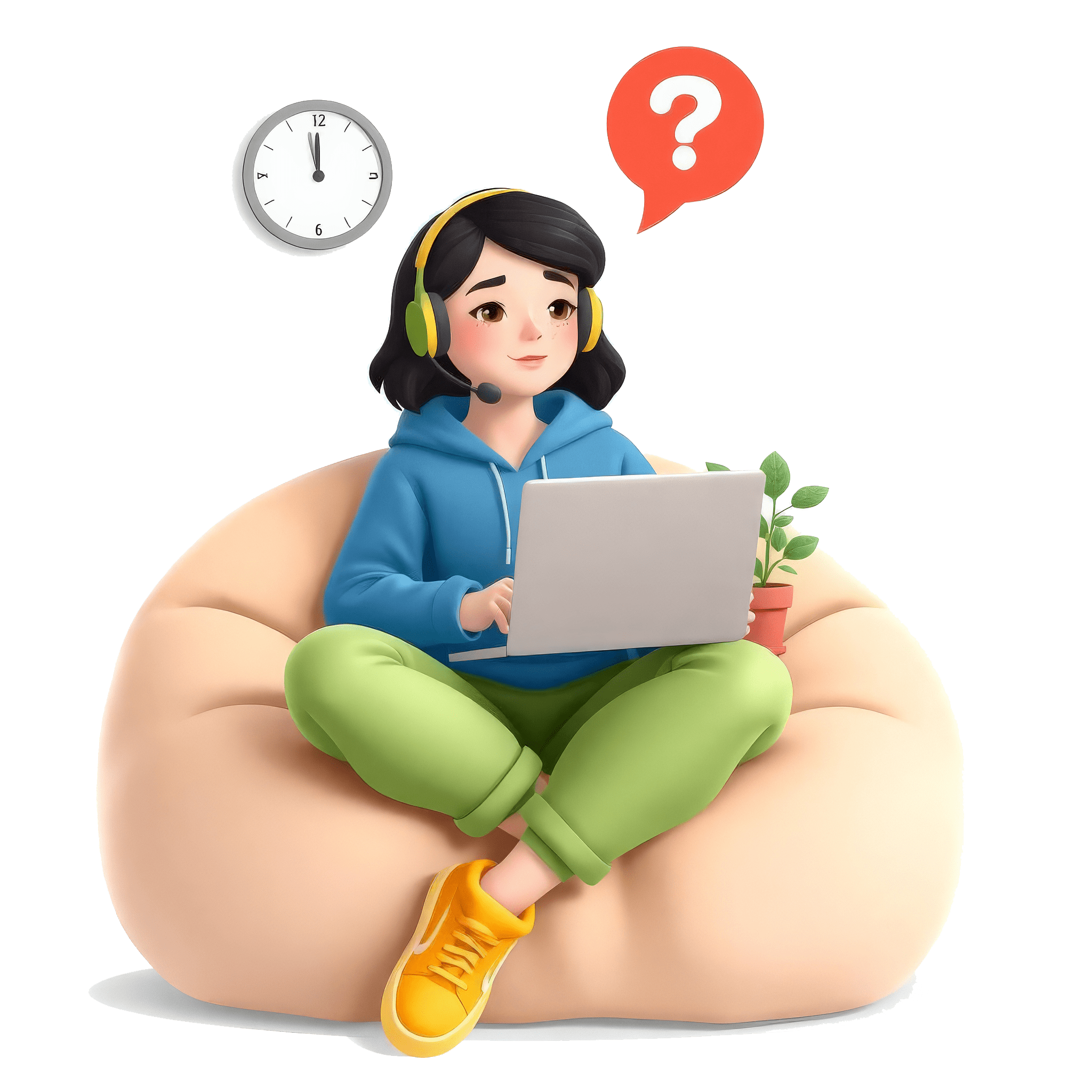
Developers & Programmers Interview
C# Question(s) & Actual Evaluation
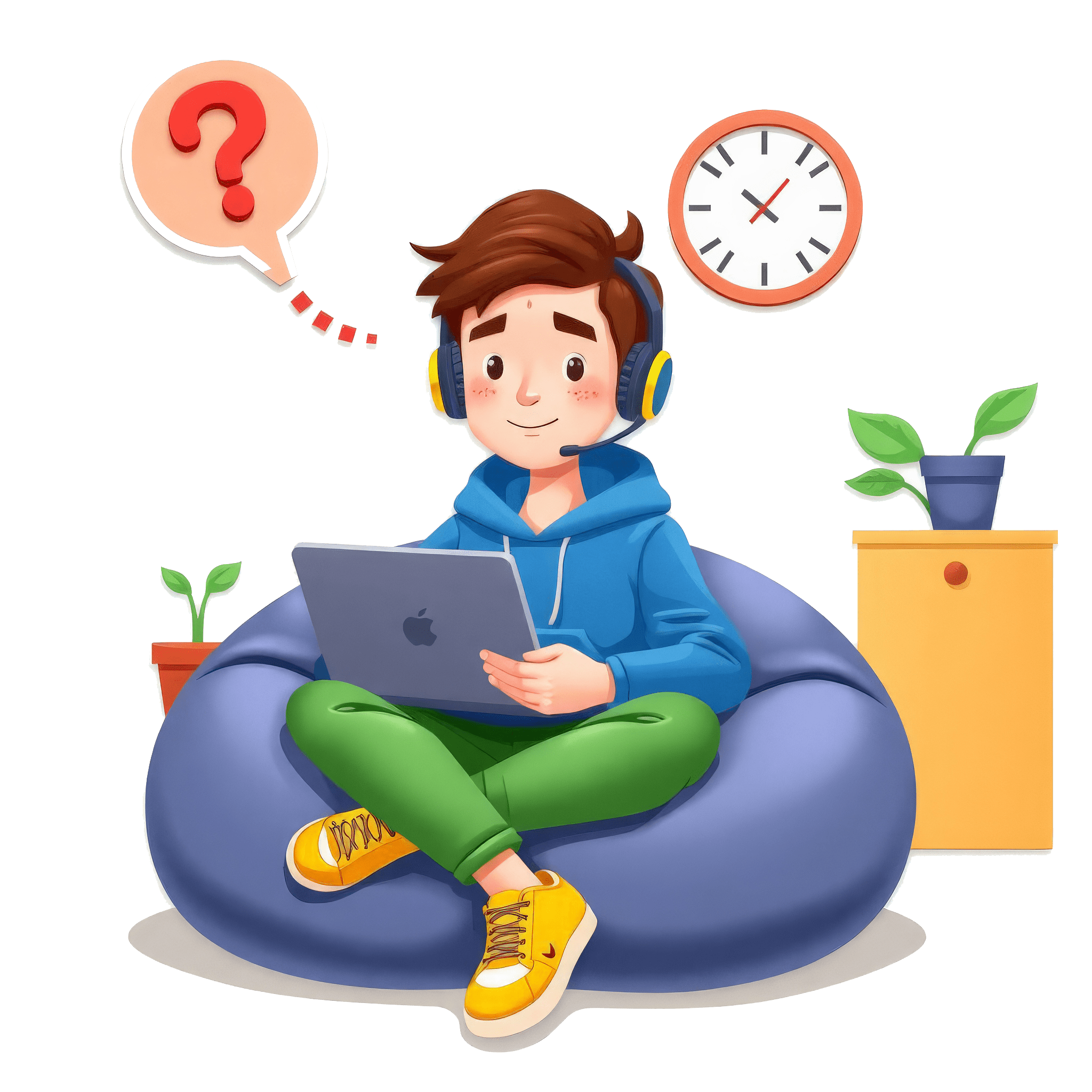
Please refer to the evaluation for more insight.
-
Classification: Developers & Programmers
-
Topic(s): C#
Can you explain the differences between value types and reference types in C#? Provide examples of each.
Candidate's Answer:The value types we can say is int value float value in plus and minus and refrence type we can say is gameobjects...
Evaluation:Your response touches on the basic idea of value types and reference types, but it lacks clarity and detail. Here’s an evaluation of your answer:
1. Correctness: You correctly identified that `int` and `float` are value types, while `GameObjects` (presumably from Unity or similar contexts) are reference types. However, you could expand on this distinction to make it more informative.
2. Clarity and Completeness: Your answer could benefit from more context. Value types store data directly, while reference types store a reference to the data's location in memory. Providing examples of both categories beyond just mentioning types (e.g., `int`, `float` for value types, and `string`, arrays, or user-defined classes for reference types) would be more comprehensive.
3. Additional Characteristics: Explaining how value types are usually allocated on the stack and have a smaller memory footprint, compared to reference types that are allocated on the heap and can have more overhead, would help solidify your answer.
Here's a refined answer you could use:
"In C#, value types and reference types differ primarily in their storage and behavior. Value types, such as `int`, `float`, and `char`, store data directly and are usually allocated on the stack. This means that when a value type is assigned to a new variable, a copy of the data is made. For example, when you declare `int a = 5; int b = a;`, `b` will have its own copy of the value `5`.
On the other hand, reference types like `string`, arrays, and class instances (such as `GameObjects` in Unity) store a reference to the data's memory location. If you assign one reference type to another, both variables point to the same data. For instance, if you declare `string str1 = "Hello"; string str2 = str1;`, modifying `str2` would not change `str1`.
In summary, value types hold their data directly, while reference types hold a reference to their data."
Rating: 2/5
To improve your answer, ensure you address key features, provide clear examples, and explain memory allocation and data handling differences between the two types. This would show a deeper understanding of the concepts.
-
Classification: Developers & Programmers
-
Topic(s): C#
What is the purpose of the `using` statement in C#, and how does it help with resource management?
Candidate's Answer:The using statement in C# is primarily used for resource management, ensuring that objects implementing the IDisposable interface are properly disposed of when they are no longer needed. This helps in managing unmanaged resources like file handles, database connections, network streams, and memory efficiently.
Evaluation:Your answer is indeed correct and captures the essence of the `using` statement in C#. You successfully highlighted its primary purpose for resource management and mentioned the importance of disposing of objects that implement the `IDisposable` interface. This is crucial when dealing with unmanaged resources, as it helps prevent memory leaks and ensures that resources are released in a timely manner.
To enhance your response, consider elaborating on a few additional points:
1. Syntax and Structure: Briefly mention the syntax of the `using` statement, such as defining the scope of the disposable object, which makes the code cleaner and easier to read.
2. Automatic Disposal: Emphasize that the `using` statement ensures that the `Dispose` method is called automatically when the code execution leaves the `using` block, simplifying resource management and reducing the risk of forgetting to explicitly call `Dispose`.
3. Code Example: Providing a simple code example could illustrate your point effectively. For instance, demonstrating a `using` block around a `StreamReader` or `SqlConnection` would solidify your understanding.
4. Performance Consideration: Mention that using the `using` statement can improve performance by ensuring that resources are released promptly, as opposed to waiting for garbage collection.
Overall, your response is solid, but incorporating these suggestions would make it even more comprehensive.
Rating: 4/5
-
Classification: Developers & Programmers
-
Topic(s): C#
Describe the concept of inheritance in C#. How does it relate to polymorphism, and could you provide a simple example?
Candidate's Answer:Inheritance in C# is a fundamental Object-Oriented Programming (OOP) concept that allows a child (derived) class to inherit properties and behaviors (methods) from a parent (base) class. It promotes code reuse and establishes a hierarchical relationship between classes. Inheritance and polymorphism go hand in hand:
Inheritance provides a way to define a general class (base class) and derive specific classes from it.
Polymorphism allows derived classes to override base class methods, providing different implementations.
Your answer is a solid start and covers the essential points of inheritance and its relationship with polymorphism in C#. You effectively describe inheritance as a mechanism for code reuse and establishing a parent-child relationship between classes. You also correctly identify that polymorphism allows derived classes to override base class methods.
To improve your answer, consider the following points:
1. Definition Clarity: While you mention that inheritance allows a derived class to inherit properties and methods, you could enhance your description by briefly explaining how this leads to reduced redundancy and easier maintenance.
2. Polymorphism Details: Expand on the concept of polymorphism by explaining its two main types: compile-time (method overloading) and run-time (method overriding). This adds depth to your explanation and clarifies how it relates to inheritance.
3. Example: Providing a simple code example would significantly strengthen your answer. Illustrating inheritance and polymorphism in action helps to contextualize these concepts for the interviewer.
4. Real-World Analogy: Including a brief analogy (e.g., car classes where "Vehicle" is the base class and "Car" and "Truck" are derived classes) can make the concepts more relatable.
Based on these suggestions, I would rate your answer a 4/5. You have a good grasp of the concepts, but incorporating a code example and some additional details would enhance clarity and comprehensiveness.