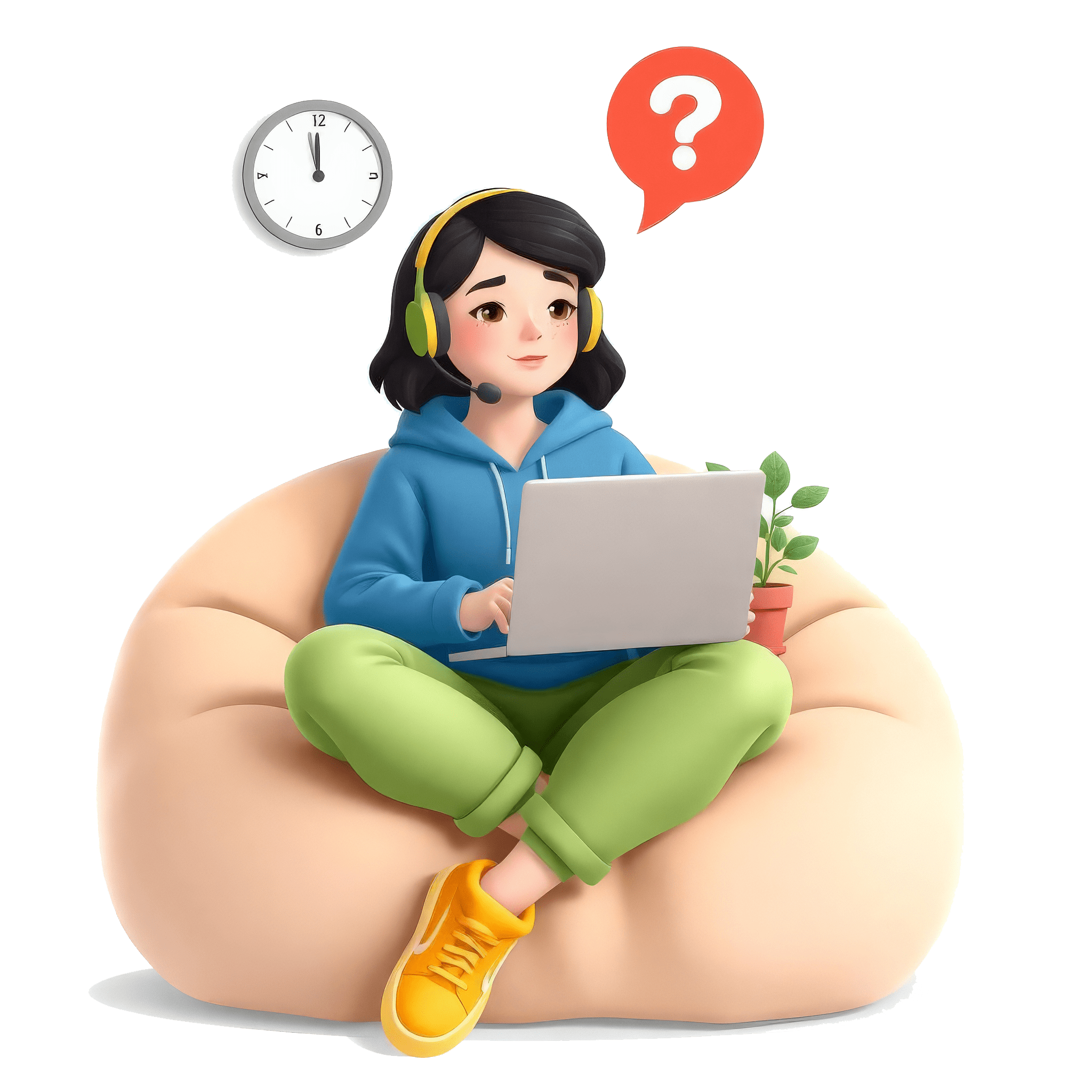
Developers & Programmers Interview
Data Structures And Algorithms Question(s) & Actual Evaluation
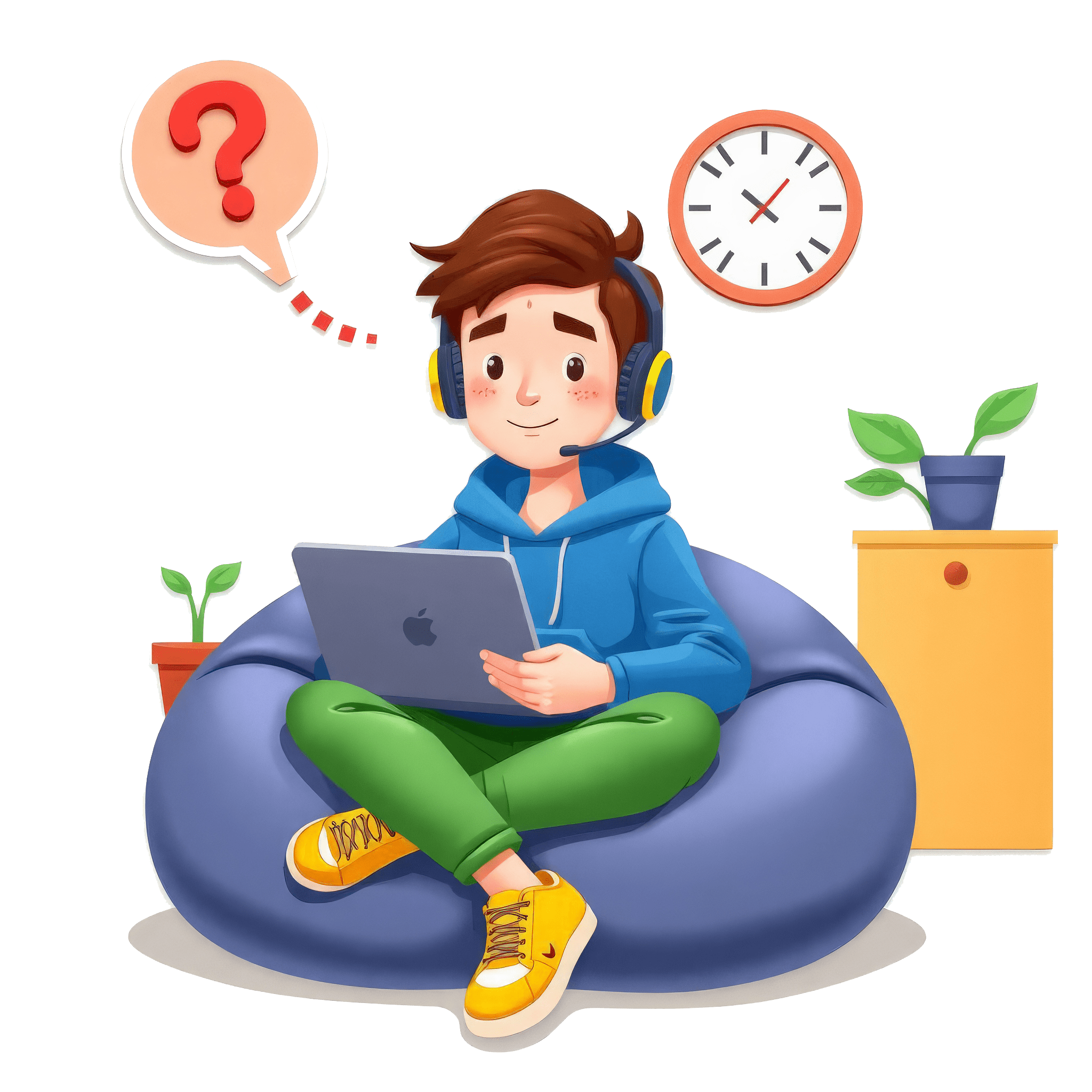
Please refer to the evaluation for more insight.
-
Classification: Developers & Programmers
-
Topic(s): Data Structures And Algorithms
Can you explain the difference between an ArrayList and a LinkedList in Java? When would you choose one over the other?
Candidate's Answer:Arraylist and Linked List both are classes of List interface. So Array list store the elements in the audit sequence and linked list also stores the elements in the.Uh, ordered sequence and umm.Yeah, that's all.
Evaluation:Your answer touches on some key points but lacks depth and clarity. While it is correct that both `ArrayList` and `LinkedList` implement the `List` interface and store elements in an ordered sequence, your response doesn't explain the fundamental differences between them or when to choose one over the other.
Key Differences:
1. Underlying Data Structure:
- `ArrayList` uses a dynamic array. Elements are stored in contiguous memory locations.
- `LinkedList` uses a doubly linked list where each element (node) contains references to the next and previous nodes.
2. Performance:
- Access Time: `ArrayList` provides O(1) time complexity for accessing elements by index due to its array-based structure. In contrast, `LinkedList` has O(n) time complexity since you might need to traverse the list to reach a specific element.
- Insertion and Deletion: `LinkedList` is more efficient with O(1) complexity for adding or removing elements when you have a reference to the node (especially at the beginning or end of the list). In `ArrayList`, these operations have O(n) complexity because elements need to be shifted.
3. Memory Usage:
- `ArrayList` may waste space if it is not full, while `LinkedList` uses more memory per element due to storage for pointers.
When to Choose:
- Choose `ArrayList` when you need fast access to elements and want to minimize memory overhead for relatively stable sizes.
- Opt for `LinkedList` when you expect a lot of insertions/deletions and do not need indexed access as frequently.
To improve your answer, focus on explaining these differences clearly and concisely. You could structure your response as follows:
"ArrayList and LinkedList are both implementations of the List interface in Java. The main difference is their underlying data structure: ArrayLists use a dynamic array, allowing O(1) access time, while LinkedLists use a doubly linked list, enabling efficient O(1) insertions and deletions. I would choose ArrayList for scenarios requiring fast random access and LinkedList for frequent modifications to the list, especially when elements are added or removed."
Rating: 2/5. You provided the basic concept but need to delve deeper into the distinctions and practical applications.
-
Classification: Developers & Programmers
-
Topic(s): Data Structures And Algorithms
Describe the time complexity of common operations (insert, delete, search) for both a hash table and a binary search tree.
Candidate's Answer:In hash table.The search takes bigger of one access time where is the any modifications like insertion or deletion might take order of north complexity, time complexity and also with binary search tree.The search would take order of N login to search a target element in the tree and insertion and insertion will take order of.Constant space order of one, whereas the deletion might take order of N time complexity.
Evaluation:Your answer contains some correct elements but also has several inaccuracies and could be clearer. Here’s a breakdown of your response and suggestions for improvement:
1. Hash Table:
- Insertion: Generally, the average case time complexity for insertion is O(1), but in the worst-case scenario (e.g., when resizing or handling hash collisions), it can degrade to O(N). Make it clear that average cases are typically O(1).
- Search: Similarly, average-case search is O(1), but worst-case can also be O(N) because of collision chaining or clustering.
- Deletion: Similar to insertion and search, average-case deletion is O(1), with O(N) in the worst case.
2. Binary Search Tree (BST):
- Insertion: Average-case time complexity for insertion is O(log N) in a balanced BST, but it can degrade to O(N) in the worst case for an unbalanced tree (e.g., a degenerate tree).
- Search: Average-case is also O(log N) for a balanced BST, with O(N) in the worst case.
- Deletion: Like insertion and search, the average case for deletion in a balanced BST is O(log N), while worst-case can reach O(N).
3. Clarity and Structure: Your answer lacks clear structure and thoroughness. State clearly each operation's time complexity for both data structures in a systematic way.
4. Terminology: Ensure you use correct terms ('order of N' should ideally be 'O(N)' and clarify 'order of one' as O(1)).
To improve your answer, structure it as follows:
- Start with the hash table, clearly stating each operation's time complexity on average and in the worst case.
- Then, follow with the binary search tree, similarly detailing the time complexities.
- Mention the importance of balance in BSTs, which affects performance.
Rating: 2/5. Your answer demonstrates some understanding, but the inaccuracies and lack of clarity hinder its effectiveness. Focus on refining your terminology and improving the structure of your response.
-
Classification: Developers & Programmers
-
Topic(s): Data Structures And Algorithms
How would you use the Java Stream API to filter a collection of objects based on specific criteria? Can you provide a simple code example?
Candidate's Answer:Filter functions takes the conditions or the predicate using lambda expression, only for the streams of objects that passes the condition will be returned. Let's take an simple example of filtering even numbers from int[]
int[] arr = {1,2,3,4,5,6}
Arrays.stream(arr).filter(i->i%2 == 0).toList();
Your answer is on the right track but could benefit from a few improvements for clarity and completeness. Here's a breakdown of your response:
1. Correctness:
- You correctly identified that the `filter` method is used to apply conditions to a stream of objects. The use of a lambda expression (`i -> i % 2 == 0`) to define the filtering criteria for even numbers is also appropriate.
2. Example Context:
- While your example is simple and demonstrates the concept of filtering, it lacks some context regarding the type of collection or data structure being used. You could specify that you are filtering an array of integers as part of a broader discussion of Java collections.
3. Comprehensiveness:
- Mentioning the import statements (like `import java.util.Arrays;`) would be helpful for someone reading your code. Additionally, explaining what the `toList()` method returns (a List of even numbers) would make your response clearer.
4. Java Stream API Context:
- It might also help to mention where the Java Stream API fits into the larger context of data structures and algorithms, specifically emphasizing its functional programming aspects and its ability to work with collections.
5. Improvements:
- You could expand your example to include how you would handle an actual collection, such as a `List<Integer>`, which is more commonly encountered than an array. Additionally, you could explain the benefits of using streams, like readability and reduced boilerplate code.
Revised Example:
```java
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(i -> i % 2 == 0)
.collect(Collectors.toList());
```
Rating: 3/5. Your answer demonstrates a basic understanding of using the Stream API for filtering, but additional context and depth would enhance it significantly.