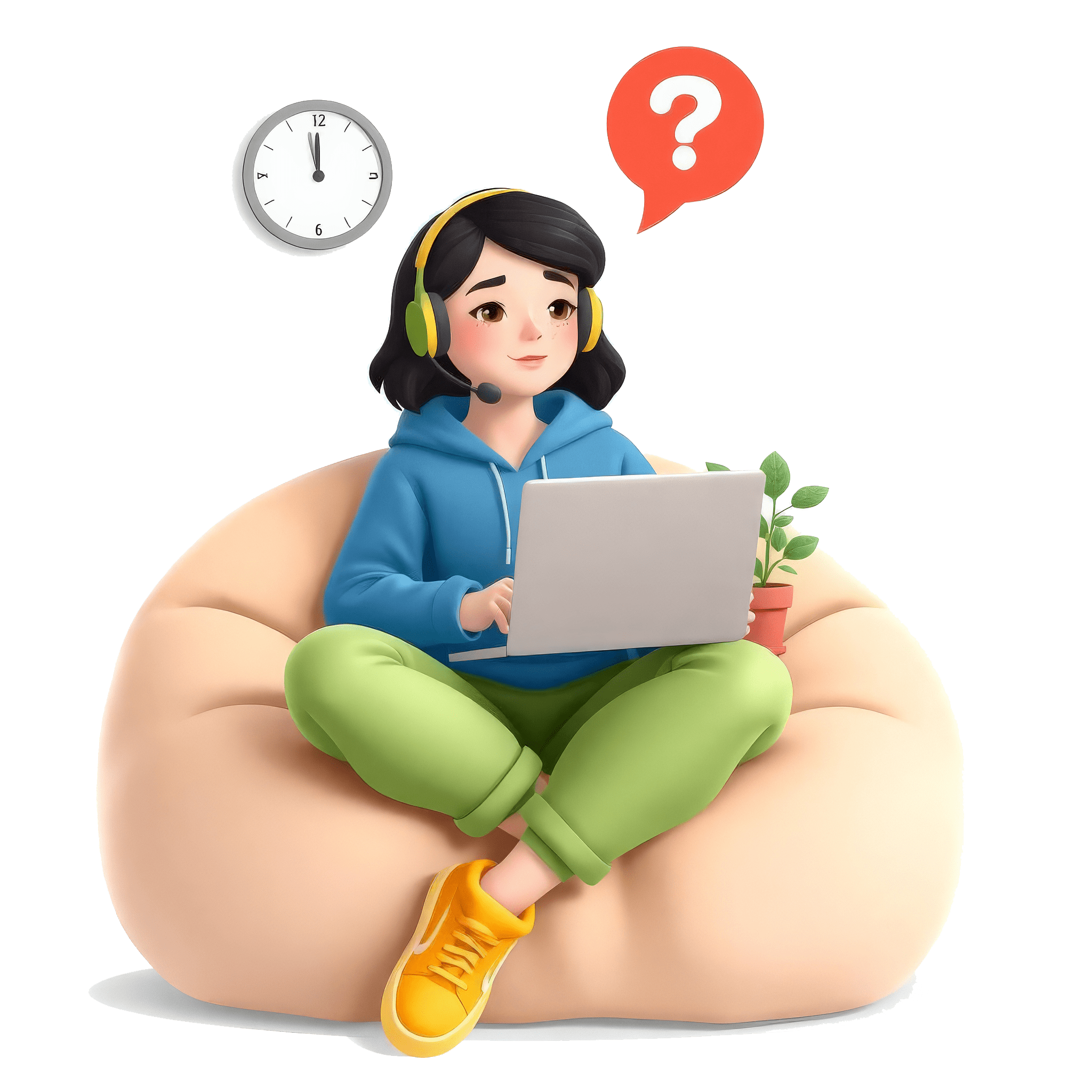
Developers & Programmers Interview
Python Question(s) & Actual Evaluation
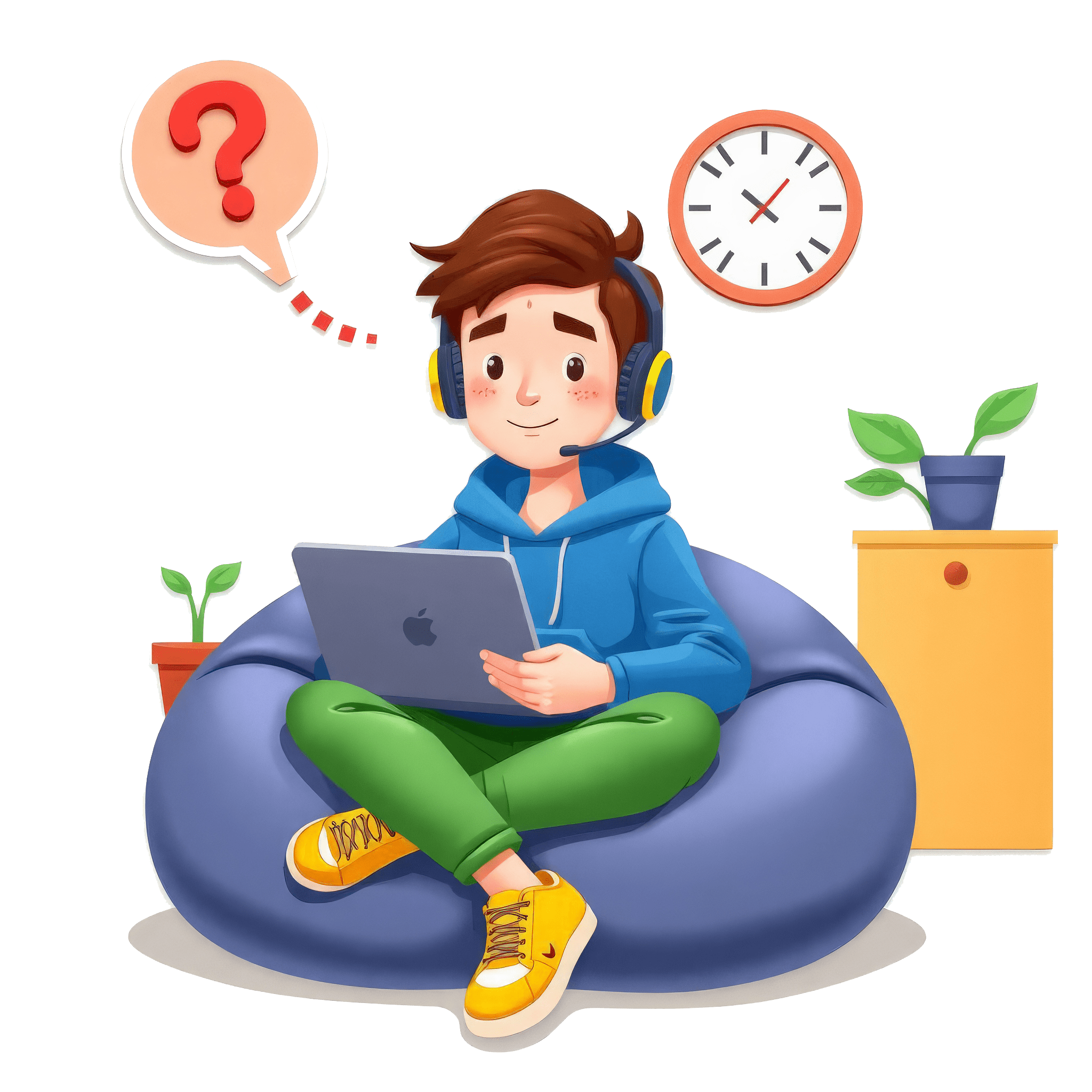
Please refer to the evaluation for more insight.
-
Classification: Developers & Programmers
-
Topic(s): Python
Can you explain the difference between a list and a tuple in Python? When would you use one over the other?
Candidate's Answer:The main difference between a least and tuple the tuples can't be changed after the created but list can be modified
Evaluation:Your answer correctly identifies the key distinction between lists and tuples: lists are mutable (modifiable), while tuples are immutable (cannot be changed after creation). This is a crucial difference to highlight and is a foundational aspect of both data structures in Python.
However, your response could benefit from additional details and clarity. Here’s a breakdown of how to enhance your answer:
1. Definition: Start with a clear definition of both lists and tuples. For example, you can define lists as ordered collections of items that can be modified, and tuples as similar collections that are fixed in size and order.
2. Use Cases: Expand on when to use one over the other. You might mention that lists are suitable for collections of items that may change throughout the program, such as a list of user inputs, while tuples are often used for fixed collections of items, such as coordinates or configuration settings.
3. Performance: You can briefly touch on performance differences; tuples are generally faster and consume less memory compared to lists, making them a better choice when creating a collection of items that won’t need modification.
4. Additional Properties: Mention that tuples can be used as keys in dictionaries due to their immutability, while lists cannot.
Improved Answer:
"The primary difference between a list and a tuple in Python is mutability. Lists are mutable, meaning their elements can be changed after creation, while tuples are immutable, meaning once they are created, they cannot be modified.
You would use a list when you need a collection of items that may change, such as a dynamic list of user inputs. On the other hand, tuples are appropriate when you have a collection of items that should not change, such as fixed coordinates or settings, and they generally have better performance due to lower memory overhead. Additionally, tuples can be used as keys in dictionaries, whereas lists cannot."
Rating: 3/5. Your original answer is on the right track but lacks depth and context. Improving it with the above points could elevate your response significantly.
-
Classification: Developers & Programmers
-
Topic(s): Python
What is a decorator in Python, and how would you use it in your code? Can you provide an example?
Candidate's Answer:A decorator in a Python is a function function that take another function and extends it behaviour without modifying its code it is applied using the decorator names in tax example
Evaluation:Your answer demonstrates a fundamental understanding of decorators in Python, but there are several areas where you could improve clarity and completeness.
First, you correctly identify that a decorator is a function that extends the behavior of another function. However, the phrasing "function function" is unclear and could be simplified to just "a function." Additionally, you mention that decorators "are applied using the decorator names in tax example," which seems to be a typographical error—perhaps you meant "syntax" instead of "tax."
To enhance your answer, you could elaborate on how decorators are used, providing a brief explanation of the syntax involved. You could also give a practical example, which would illustrate your understanding more effectively. Including practical applications of decorators (like logging, enforcing access control, or modifying input/output) would further demonstrate their utility.
Here’s an improved version of your answer:
"A decorator in Python is a function that takes another function as an argument and extends its behavior without modifying the original function's code. Decorators are applied using the `@decorator_name` syntax directly above the function definition.
For instance, let's say we want to log the execution time of a function. We can create a decorator to achieve that:
```python
import time
def timer_decorator(func):
def wrapper(*args, kwargs):
start_time = time.time()
result = func(*args, kwargs)
end_time = time.time()
print(f'Execution time: {end_time - start_time:.4f} seconds')
return result
return wrapper
@timer_decorator
def say_hello(name):
time.sleep(1) # Simulating a time-consuming task
print(f'Hello, {name}!')
say_hello('Alice')
```
In this example, `timer_decorator` wraps the `say_hello` function, measuring and printing its execution time."
Rating: 3/5. Your response had the right idea, but clarity and specificity were lacking.
-
Classification: Developers & Programmers
-
Topic(s): Python
Describe a time you encountered a significant bug in your code. How did you diagnose and fix the issue?
Candidate's Answer:Seeing the function or a program to evaluate the work according to the program how it look like
Evaluation:Your answer lacks clarity and specific detail, which makes it difficult to understand how you addressed the bug. It seems you intended to convey a process of evaluating the function or program, but without a structured explanation, it's hard for the interviewer to grasp the full scope of your experience.
To improve your answer, consider using the STAR method (Situation, Task, Action, Result) to provide a clear narrative:
1. Situation: Briefly describe the context in which you encountered the bug.
2. Task: Specify your role and responsibility in this situation.
3. Action: Detail the steps you took to diagnose and fix the bug, such as using debugging tools, adding logging, testing hypotheses, or consulting documentation.
4. Result: Share the outcome after you fixed the bug, highlighting how it improved your code, enhanced performance, or helped your team.
For example, you might say, "In one project, I encountered a bug where the program was returning unexpected results. As the lead developer, I used print statements and logging to trace the issue, which led me to discover that a logical error in a loop was causing the problem. After making the corrections, I tested the function thoroughly and confirmed it returned the expected output. This fix not only resolved the immediate issue but also improved the code's overall performance."
By providing this structured response, you demonstrate problem-solving skills and a systematic approach to debugging.
Rating: 1/5. Your answer needs significant improvement in clarity and detail.